Quickstart
This guide will get you all set up and ready to use adCAPTCHA. We’ll cover integration with both the backend and frontend, creating an end-to-end solution. This guide covers the JavaScript language, but the integration process is the same regardless of which languages you use.
Before you can follow this guide, you will need an adCAPTCHA account. Please reach out to our sales team to get started here.
Placement ID and API Key
As a bare minimum, you will need a Placement ID and an API Key to get started with adCAPTCHA. These are both found in the adCAPTCHA dashboard.
Placement ID
The Placement ID is a unique identifier for your adCAPTCHA placement. It gives you control over the appearance and behavior of the adCAPTCHA widget. You are free to create as many placements as you like, it's common for websites to have multiple places that the CAPTCHA should appear but have all of them use the same Placement ID.
Step 2
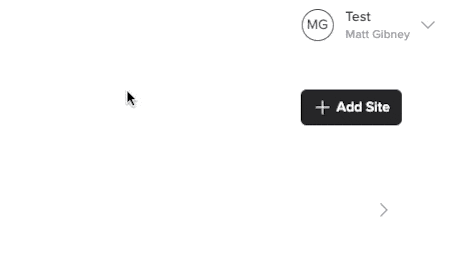
Create a new Site. A site in the adCAPTCHA dashboard represents a website where you want to use adCAPTCHA.
Step 3
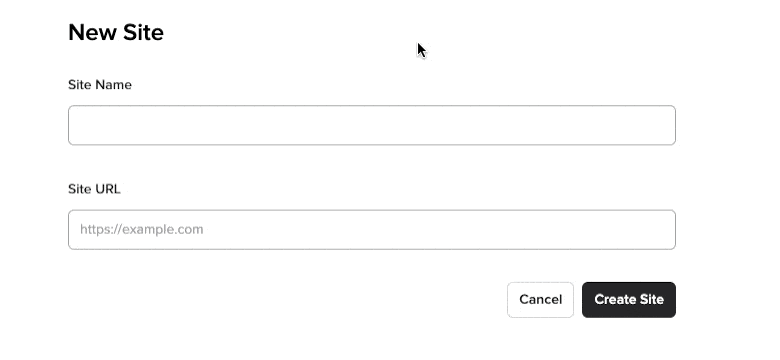
Sites need a name and a URL. The URL must be a valid TLD and must be HTTPS. We cannot support HTTP URLs for security reasons.
Step 4
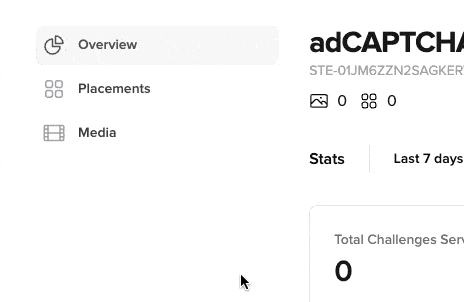
Select the Placements tab in the site navigation. Here you can create a new placement for your site.
Step 5
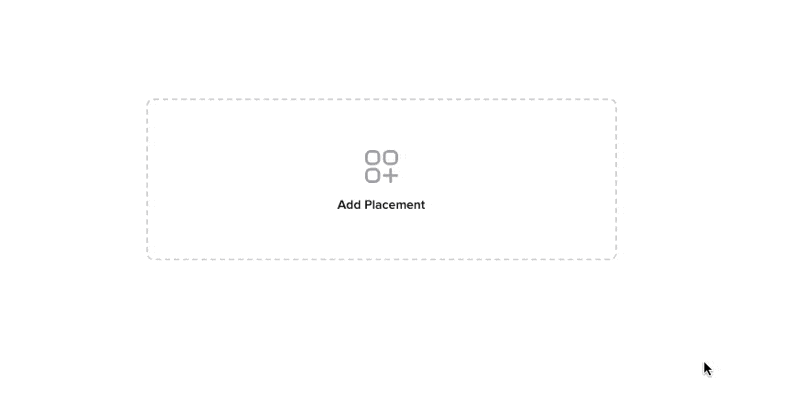
Create a new Placement. Each placement needs a name, this is only used internally and does not affect adCAPTCHA at all. Once created, you will be given a Placement ID.
API Key
The API Key is used to authenticate your requests to the adCAPTCHA API. It is a secret key that should be kept secure and not shared with anyone. Do not expose your API Key in public repositories or client-side code. If you suspect your API Key has been compromised, you can regenerate it in the adCAPTCHA dashboard.
Step 1
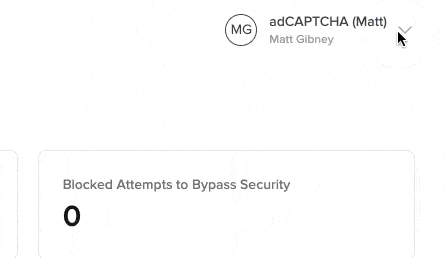
Open the My Account page witht he dropdown menu in the top right corner of the adCAPTCHA dashboard.
Step 3
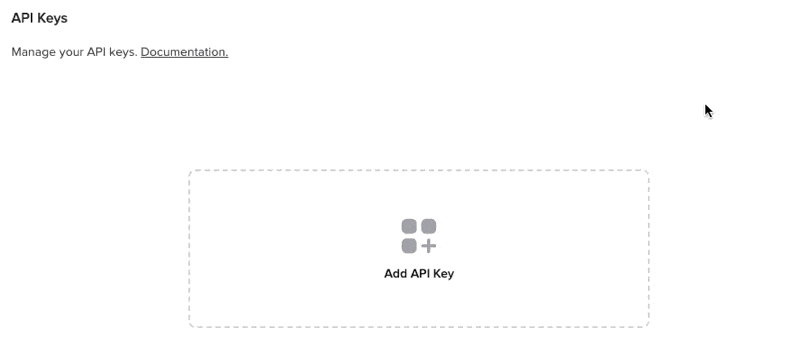
Create a new API Key. Each key needs a name, this is only used internally and does not affect adCAPTCHA at all. Once created, you will be given an API Key. This is the only time you will see the key, so make sure to copy it somewhere safe.
It is possible to have more than one API key available in your account. This can be useful if you want to have different keys for different environments, such as development, staging, and production.
It's also useful if you would like to employ a best prectise of rotating your API keys periodically. This can be done by creating a new key and updating your application to use the new key before disabling the old one.
Frontend Integration
There are multiple ways to approach the fromtend integration of adCAPTCHA. Before proceeding, we recommend checking out the Integrations list to see if we have a pre-built integration for your platform.
We won't be using one of the pre-built integrations in this guide, but we will show you how to integrate adCAPTCHA into your website using plain JavaScript.
Adding our script to your pages
Firstly, the adCAPTCHA integration needs to be added to your website. We strongly recommend that this be added to the head for the simplest experience.
adCAPTCHA Frontend Widget
<script src="https://widget.adcaptcha.com/index.js" defer></script>
The script is served by AWS CloudFront and is distributed globally for fast load-time. We strongly suggest using defer, as per our example above, to ensure that the loading of the script does not impact rendering time.
Adding Triggers
We give you full control of where you'd like CAPTCHA triggers to appear on your page. You can also place as many as you'd like (This is useful in cases where you may have multiple forms on a single page). By default, the adCAPTCHA widget will inject a trigger into any div
that has a data-adcaptcha
attribute.
adCAPTCHA Trigger
<div data-adcaptcha="PLACEMENT_ID" />
The value of the attribute is called a placementID
. Placement IDs are generated by AdCaptcha and a valid placement ID is required for CAPTCHA challenges to work. When placing multiple triggers on the page, you can use the same placementID
or different ids for each. The only benefit to be gained from using different IDs is currently for analytics purposes. During the integration process, we will generate these placement IDs for you.
Initialisation
After the page has loaded, you should initialise the adCAPTCHA widget by calling window.adcap.init()
. This will prepare the widget and add CAPTCHA triggers wherever you have specified. The init
function MUST be called for adCAPTCHA to work, we recommend calling it after the page has finished loading.
window.adcap.init();
There are optional configurations that can be passed to the init function, such as:
Option | Description |
---|---|
triggerSelector | This attaches a selector to the trigger element of your choice. |
language | This sets the language of the trigger and CAPTCHA. By default, the language is changed to the user's browser language, but if not supported, it will default to English. |
analyticsEnabled | This enables or disables collecting analytics data for the CAPTCHA. By default, it is enabled. |
Supported Languages
en
- Englishit
- Italianes
- Spanish
Handling on complete
It’s very likely that you will want to execute some code after the user has successfully completed the CAPTCHA challenge. The most common use case is to remove the disabled attribute from submission buttons and change styling rules.
You can specify a callback diring init
or after the widget has been initialised using the setupTriggers
function. This can be called at any time, which is useful if you have dynamic forms on your page. Here is an example of this being used:
window.adcap.init({
onComplete: () => {
console.log('CAPTCHA Completed');
}
});
Verify Success Token
The most vital part of the adCAPTCHA integration is the backend verification. This is where you will verify the user's response to the adCAPTCHA challenge.
You can retrieve the sucess token from window.adcap.successToken
Attributes
- Name
token
- Type
- string
- Description
The success token your website received after the user successfully completed their challenge.
Potential Errors
- Name
400
- Type
- string
- Description
Token missing
- Name
400
- Type
- string
- Description
Invalid token
- Name
400
- Type
- string
- Description
Token already verified
Request
curl -X POST "https://api.adcaptcha.com/v1/verify" \
-H "Authorization: Bearer 01H70KCBK6ZWMER7RVJMB4YW78" \
-H "Content-Type: application/json; charset=utf-8" \
-d '{"token":"eyJhbGciOiJIUzI1NiIsInR5cCI6IkpXVCJ9.eyJjaGFsbGVuZ2VJbnN0YW5jZUlEIjoiQ0hJLTAxSEFQSFpUWVRaOTEyMUYyRkU3TlZTNEFTIiwicGxhY2VtZW5"}'
Response
{ "message":"Token verified" }
What's next?
Great, you're now set up with a working adCAPTCHA integration. It's using default media and settings, but you can customise the appearance and behaviour of the adCAPTCHA widget to suit your website. Here are some next steps you might want to take: